Batch update: Como fazer atualização em lote em Java:
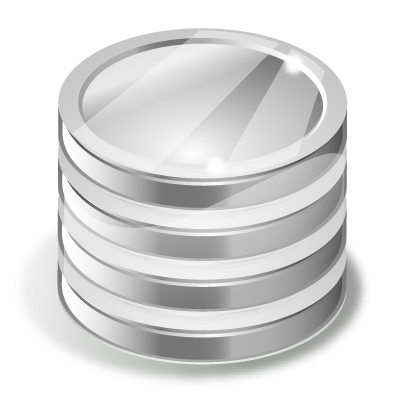
Warning: WP_Syntax::substituteToken(): Argument #1 ($match) must be passed by reference, value given in /home/feltexco/public_html/felix/wp-content/plugins/wp-syntax/wp-syntax.php on line 380
Warning: WP_Syntax::substituteToken(): Argument #1 ($match) must be passed by reference, value given in /home/feltexco/public_html/felix/wp-content/plugins/wp-syntax/wp-syntax.php on line 380
Warning: WP_Syntax::substituteToken(): Argument #1 ($match) must be passed by reference, value given in /home/feltexco/public_html/felix/wp-content/plugins/wp-syntax/wp-syntax.php on line 380
Como fazer atualização em lote em Java: batch update
Olá Amigos, hoje iremos mostrar como podemos fazer atualização em Lote no Java o chamado batch update. Para isso precisaremos criar uma massa de teste com 10000 registros. Em seguida será feita uma inserção via JDBC utilizando Insert normalmente e outra vez utilizando a abordagem batch update. Veja a seguir os resultados que tivemos batch update X Insert simples.
1. Criando a tabela do banco de dados
Segue o script para a criação da tabela.
1 2 3 4 5 6 | CREATE TABLE tbaluno ( matricula INT NOT NULL , nome VARCHAR(45) NULL , telefone VARCHAR(45) NULL , email VARCHAR(45) NULL , datacadastro DATETIME NULL ); |
Atenção: não foi criada a chave primária para evitar conflito na geração das chaves pelo programa!
2. Construção da classe de modelo
Inicialmente devemos criar a classe Aluno conforme código abaixo.
Ela servirá como modelo de negócio para atualizarmos a tabela no banco de dados.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 | package br.com.feltex.jdbc; import java.io.Serializable; import java.util.Date; public class Aluno implements Serializable { private static final long serialVersionUID = -309513637403441918L; private Long matricula; private String nome; private String telefone; private String email; private String endereco; private Date dataCadastro; public Aluno() { } public Aluno(Long matricula) { super(); this.matricula = matricula; } public Aluno(Long matricula, String nome) { super(); this.matricula = matricula; this.nome = nome; } public Date getDataCadastro() { return dataCadastro; } public String getEmail() { return email; } public String getEndereco() { return endereco; } public Long getMatricula() { return matricula; } public String getNome() { return nome; } public String getTelefone() { return telefone; } public void setDataCadastro(Date dataCadastro) { this.dataCadastro = dataCadastro; } public void setEmail(String email) { this.email = email; } public void setEndereco(String endereco) { this.endereco = endereco; } public void setMatricula(Long matricula) { this.matricula = matricula; } public void setNome(String nome) { this.nome = nome; } public void setTelefone(String telefone) { this.telefone = telefone; } @Override public String toString() { return "Aluno [matricula=" + matricula + ", nome=" + nome + ", telefone=" + telefone + ", email=" + email + ", dataCadastro=" + dataCadastro + "]"; } } |
3. A classe que realiza as operações
A seguir teremos a classe principal que executará as ações no banco de dados e fará a comparação entre os tempos gastos entre as operações Uma a uma e tipo de atualização via lote.
Temos o método “gerarMassa” que criará uma coleção de alunos que será inserido no Banco de dados. O método “executarBatch” faz as inserções dos dados em blocos. Temos também o “executarUnitario” que faz as inserções item por item. Há também o método “getConexao” que recupera um conexão com o banco de Dados. Por último, mas não menos importante, há o método “main” que inicia a aplicação.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 | package br.com.feltex.jdbc.avancado; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.util.ArrayList; import java.util.Date; import java.util.List; import br.com.feltex.jdbc.Aluno; public class Transacao { public static void gerarMassa(){ for (int i = 0; i < 10000; i++) { Aluno aluno = new Aluno(); aluno.setMatricula(Long.valueOf(i)); aluno.setNome("Jose" + i); aluno.setTelefone("22352-" + i); aluno.setEmail("Jose" + i + "@feltex.com.br"); aluno.setEndereco("RUA a Numero: " + i); aluno.setDataCadastro(new Date()); listaAlunos.add(aluno); } } static List<Aluno> listaAlunos = new ArrayList<>(); public static void executarBatch() { Long inicio = System.currentTimeMillis(); Long fim = 0L; Connection conexao = null; try { conexao = getConexao(); conexao.setAutoCommit(false); PreparedStatement pstm = conexao .prepareStatement("Insert into tbaluno (matricula, nome," + " telefone, email, datacadastro) values (?,?,?,?,?)"); int contador = 0; for (Aluno aluno : listaAlunos) { contador++; pstm.setLong(1, aluno.getMatricula()); pstm.setString(2, aluno.getNome()); pstm.setString(3, aluno.getTelefone()); pstm.setString(4, aluno.getEmail()); pstm.setDate(5, new java.sql.Date(aluno.getDataCadastro() .getTime())); pstm.addBatch(); } if ((contador % 100) == 0) { pstm.executeBatch(); } conexao.commit(); pstm.close(); conexao.close(); fim = System.currentTimeMillis(); } catch (Exception e) { try { conexao.rollback(); } catch (Exception e2) { e.printStackTrace(); } e.printStackTrace(); } System.out.println("executarBatch Tempo total[ms]: " + (fim - inicio)); } public static void executarUnitario() { Long inicio = System.currentTimeMillis(); Long fim = 0L; Connection conexao = null; try { conexao = getConexao(); PreparedStatement pstm = conexao .prepareStatement("Insert into tbaluno (matricula, nome," + " telefone, email, datacadastro) values (?,?,?,?,?)"); for (Aluno aluno : listaAlunos) { pstm.setLong(1, aluno.getMatricula()); pstm.setString(2, aluno.getNome()); pstm.setString(3, aluno.getTelefone()); pstm.setString(4, aluno.getEmail()); pstm.setDate(5, new java.sql.Date(aluno.getDataCadastro() .getTime())); pstm.execute(); } pstm.close(); conexao.close(); fim = System.currentTimeMillis(); } catch (Exception e) { e.printStackTrace(); } System.out.println("executarUnitario Tempo total [ms]: " + (fim - inicio)); } /** * * @return */ public static Connection getConexao() { //TODO Remover este código desta classe Connection conexao = null; String usuario = "root"; String senha = "root"; String nomeBancoDados = "bdacademicnet"; try { conexao = DriverManager.getConnection( "jdbc:mysql://localhost:3306/" + nomeBancoDados, usuario, senha); } catch (Exception e) { e.printStackTrace(); } return conexao; } public static void main(String[] args) { gerarMassa(); for (int i = 0; i < 10; i++) { executarUnitario(); executarBatch(); } } } |
A saída deve ser parecida com a imagem abaixo:
4. Conclusão
A execução desde exemplo nos mostra qual a vantagem de utilizamos de utilizar a gravação em lotes quando temos grandes volumes de dados. Observe que o resultados são bem diferentes. Quando utilizamos a gravação batch update com um volume considerável de dados temos um ganho de desempenho.
Isto é apenas o início. Aproveite para ler os links relacionados abaixo e buscar mais informações sobre o assunto.
Então é isso. Por hoje é só e vida que segue.
Deixe um comentário
Você precisa fazer o login para publicar um comentário.